Its Simple way to enable the Datepicker when Click the text field in PHP Yii Frameworks.
Step 1 - Install the Datepicker widget to the yii-2 with the help of procedures in the following link.
https://github.com/2amigos/yii2-date-picker-widget
Step 2 - Remove the Textfield code from the form.php
<?= $form->field($model, 'date')->textInput(['maxlength' => true]) ?>
Step 3 - Replace the textfield code by the follwing
Step 1 - Install the Datepicker widget to the yii-2 with the help of procedures in the following link.
https://github.com/2amigos/yii2-date-picker-widget
Step 2 - Remove the Textfield code from the form.php
<?= $form->field($model, 'date')->widget ( DatePicker::className(), [ // inline too, not bad 'inline' => false, // modify template for custom rendering 'clientOptions' => [ 'autoclose' => true, 'format' => 'dd-M-yyyy'] ] ); ?>
Step 4 - Have to access the Datepicker at the beginning , just copy the following and paste it.
use dosamigos\datepicker\DatePicker ;
Output -
Before Clicking the text field
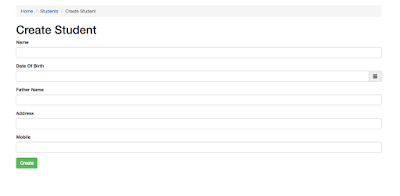
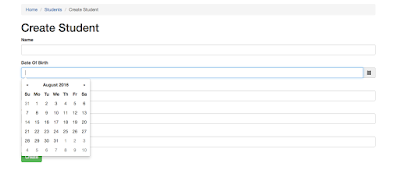